Chat Widget
The ConnectyCube Web Chat Widget is designed to simplify the process of adding chat functionality to your Web apps. This widget offers an out-of-the-box solution for embedding chat features - such as instant messaging, user presence, and file sharing - without the overhead of building a complete chat system from scratch. Key benefits include:
- Easy integration: plug the widget into your existing Web projects.
- Customizable interface: adjust the look and feel to match your brand.
- Real-time messaging: leverage ConnectyCube’s reliable backend for instant communication.
- Responsive design: works seamlessly on both desktop and mobile devices.
- Modular and extensible: adapt the widget to your unique requirements.
Split-view mode
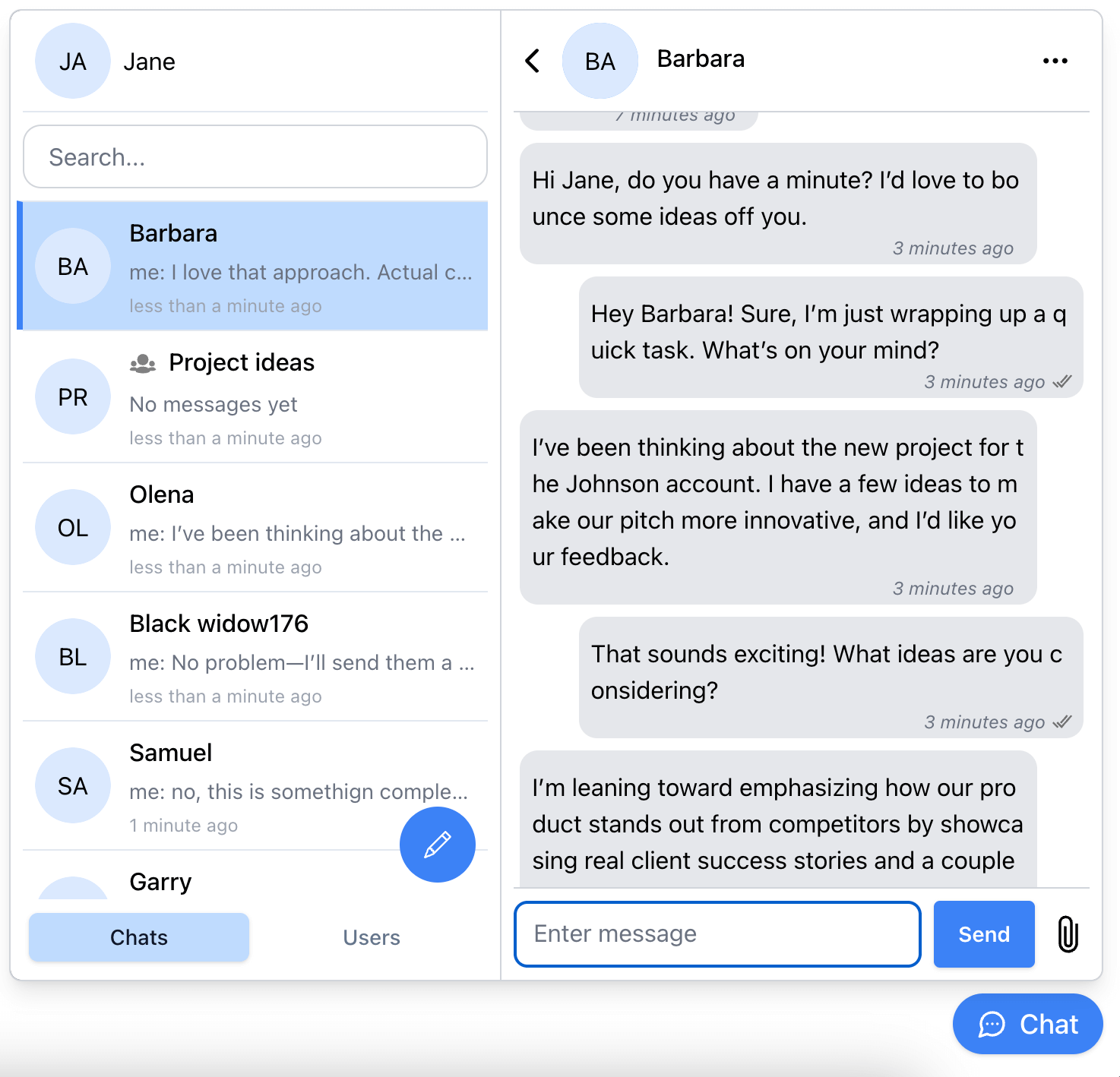
Single-view mode
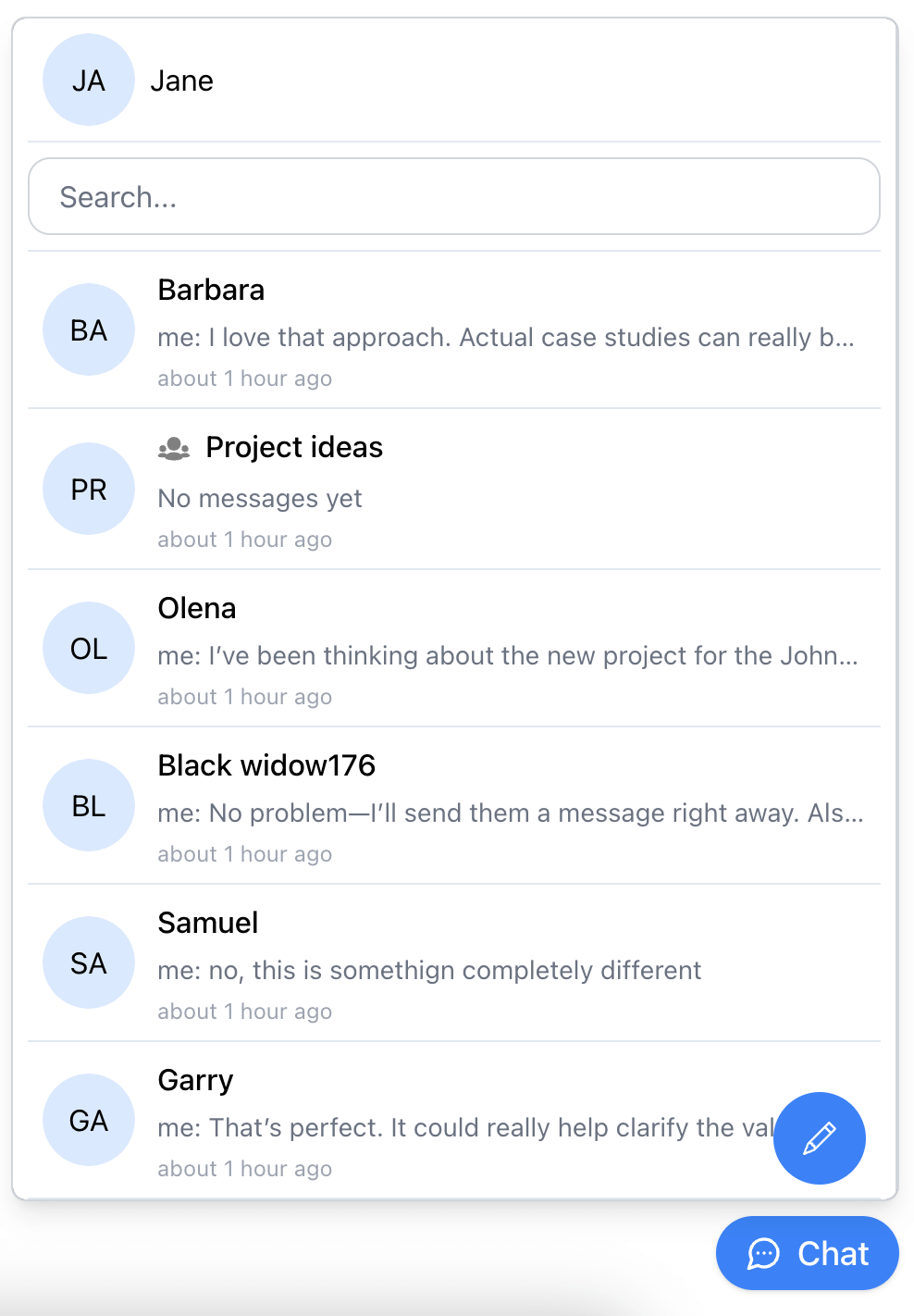
Code samples
Section titled “Code samples”See chat widget code samples as a reference for faster integration.
Installation
Section titled “Installation”npm install @connectycube/chat-widget
// or
yarn add @connectycube/chat-widget
Add the following scripts on your html page somewhere in head
element:
<script src="https://unpkg.com/react@18/umd/react.production.min.js" crossorigin></script><script src="https://unpkg.com/react-dom@18/umd/react-dom.production.min.js" crossorigin></script><script src="https://unpkg.com/connectycube@4/dist/connectycube.min.js"></script><script src="https://unpkg.com/@connectycube/chat-widget@latest/dist/index.umd.js"></script>
Display widget
Section titled “Display widget”Before you start
Section titled “Before you start”Before you start, make sure:
- You have access to your ConnectyCube account. If you don’t have an account, sign up here.
- An app created in ConnectyCube dashboard. Once logged into your ConnectyCube account, create a new application and make a note of the app credentials (App ID and Auth Key) that you’ll need for authentication.
Import and place the following component in your app:
import ConnectyCubeChatWidget from "@connectycube/chat-widget";
...
<ConnectyCubeChatWidget appId="123" authKey="11111111-2222-3333-4444-55555555" userId="45" userName="Samuel" showOnlineUsersTab={false} splitView={true}/>
// userName - how other users will see your user name// userId - a User Id from your system
See chat widget code samples as a reference for faster integration.
React 19
Section titled “React 19”By default, the widget uses the React 18-compatible build. To use it with React 19, import the dedicated ESM-only build instead:
import ConnectyCubeChatWidget from '@connectycube/chat-widget/react19';
Vanilla JS
Section titled “Vanilla JS”Place the following script in your app:
<!doctype html><html lang="en"> <head> <!-- ... --> </head> <body> <!-- ... --> <!-- @connectycube/chat-widget - start --> <script src="https://unpkg.com/react@18/umd/react.production.min.js" crossorigin></script> <script src="https://unpkg.com/react-dom@18/umd/react-dom.production.min.js" crossorigin></script> <script src="https://unpkg.com/connectycube@4/dist/connectycube.min.js"></script> <script src="https://unpkg.com/@connectycube/chat-widget@latest/dist/index.umd.js"></script> <script> const chatWidgetContainer = document.createElement('div'); chatWidgetContainer.id = 'ConnectyCube_chat-widget'; document.body.appendChild(chatWidgetContainer); const props = { appId: 123, authKey: '11111111-2222-3333-4444-55555555', userId: '45', // a User Id from your system userName: 'Samuel', // how other users will see your user name showOnlineUsersTab: false, splitView: true, }; ReactDOM.createRoot(chatWidgetContainer).render(React.createElement(ConnectyCubeChatWidget, props)); </script> <!-- @connectycube/chat-widget - end --> </body></html>
See chat widget code samples as a reference for faster integration.
Feature configuration
Section titled “Feature configuration”This section explains how to control specific features of the chat widget by enabling or disabling them through configuration props. Customize your widget behavior to meet your specific requirements without having to modify the core code.
Notifications
Section titled “Notifications”This section allows you to configure how users are notified about new messages. You can control notifications, browser push notifications, and notification sounds independently for a fully customizable experience:
- showNotifications - show browser notifications about new messages received
- webPushNotifications - show browser push notifications about new messages received
- playSound - play sound about new messages received
Online users
Section titled “Online users”showOnlineUsersTab - displays a dedicated tab within the chat widget that lists all currently online users. This feature is useful in team or community-based chat environments where it’s helpful to see who’s available before starting a conversation.
Toggle Button visibility
Section titled “Toggle Button visibility”hideWidgetButton - controls the visibility of the floating button that users click to open or close the chat widget. This is useful when you want to launch the widget programmatically or embed it inside a custom UI element instead of showing the default button. Read how to open a widget by clicking on custom button
New chat buttons
Section titled “New chat buttons”These settings let you control whether users can start new chats or initiate one-on-one conversations. Use them to restrict chat creation in specific environments or use cases, such as read-only communities or customer support-only contexts.
- hideNewChatButton - controls the visibility of the main “New Chat” button in the chat widget interface.
- hideNewUserChatOption - hides the option to start a new 1-on-1 conversation from within the “Create Chat” flow. This is useful when you only want to allow group chats or predefined channels.
Moderation
Section titled “Moderation”The moderation settings help maintain a safe and respectful chat environment. These options allow users to report inappropriate content and manage their personal block lists, giving them more control over their experience.
At then moment the following moderation features supported:
- enableContentReporting - users can report messages via a UI action
- enableBlockList - users can block and unblock others from their profile
Report user
Section titled “Report user”To enable Report user feature, you need to pass enableContentReporting: true
prop. This will display a Report button in user profile:
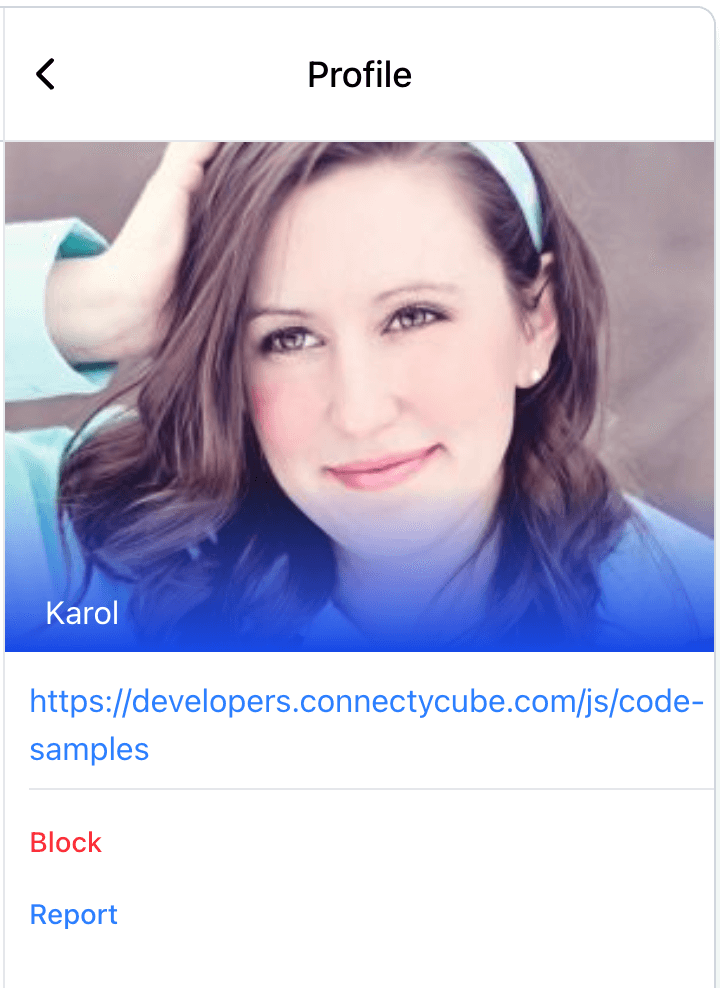
For user reporting to work properly, it requires the following:
- Go to ConnectyCube Daashboard
- select your Application
- Navigate to Custom module via left sidebar
- Create new table called UserReports with the following fields:
- reportedUserId - integer
- reason - string
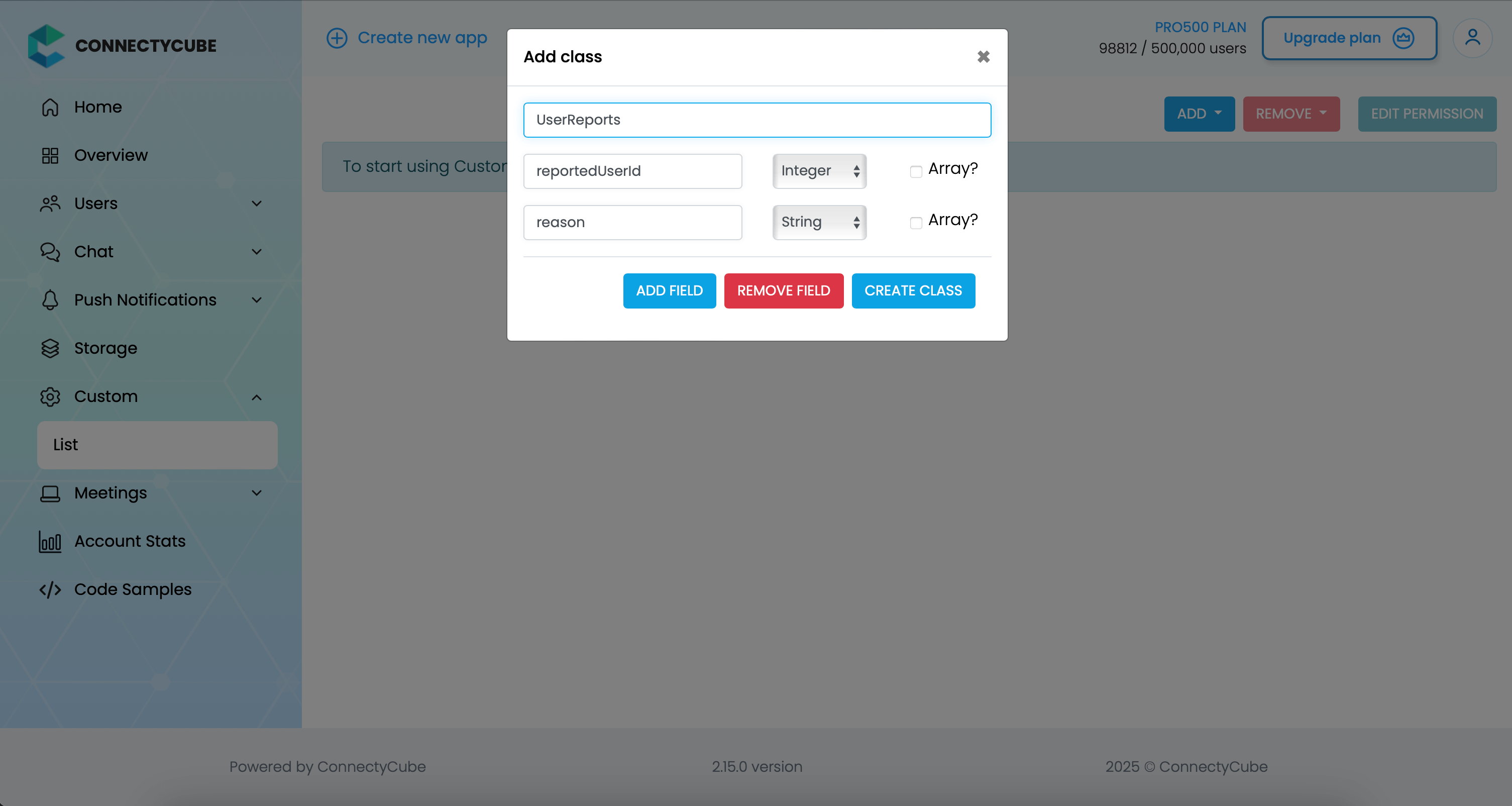
Once the table is created, you can test the user reporting in Widget. You will see the reports appear in this table.
Block user
Section titled “Block user”To enable Block user feature, you need to pass enableBlockList: true
prop. This will display a Block button in user profile (see above).
Appearance & Styling
Section titled “Appearance & Styling”The Appearance & Styling settings let you fully customize the visual presentation of the chat widget. You can control the look of the toggle button, badge, and chat portal using inline styles or CSS class names. This ensures your widget blends seamlessly into your app or site design.
Chat toggle button
Section titled “Chat toggle button”- buttonTitle - sets the accessible title (title attribute) of the chat toggle button. This is typically used for accessibility or tooltip text when hovering over the button
- buttonStyle - applies inline styles to the chat toggle button
Portal
Section titled “Portal”- portalStyle - applies inline styles to the main chat window (portal)
- badgeStyle - applies inline styles to the notification badge that appears on the toggle button (e.g., unread messages count)
Prop Name | Type | Description | Default Value |
---|---|---|---|
appId | number | string | The ConnectyCube Application ID | |
authKey | string | The ConnectyCube Authentication Key | |
config | object | (Optional) Configuration options for ConnectyCube SDK | |
userId | string | A User Id from your system | |
userName | string | User name. This is how other users will see your user name | |
userAvatar | string | (Optional) User Avatar URL | |
userProfileLink | string | (Optional) User profile link URL | |
translation | string | (Optional) Specifies the language for the chat widget. See Supported Translations | ”en” |
disableClickOutside | boolean | (Optional) Hide chat widget by click or touch outside the widget’s containers | false |
disableEscKeyPress | boolean | (Optional) Hide chat widget by press “Escape” button | false |
hideWidgetButton | boolean | (Optional) Allows to hide the button that opens/hides the chat widget | false |
buttonTitle | string | (Optional) The text displayed on the chat button | ”Chat” |
portalStyle | React.CSSProperties | (Optional) Inline styles for the portal | |
overlayStyle | React.CSSProperties | (Optional) Inline styles for the overlay | |
buttonStyle | React.CSSProperties | (Optional) Inline styles for the button | |
badgeStyle | React.CSSProperties | (Optional) Inline styles for the unread messages badge | |
onlineBadgeStyle | React.CSSProperties | (Optional) Inline styles for the online users count badge | |
open | boolean | (Optional) To control the visibility state of the chat widget | false |
embedView | boolean | (Optional) Embeds chat view by filling parent block. Props open and hideNewChatButton won’t react and will be true by default | false |
splitView | boolean | (Optional) Displays the chats in split view or single view | false |
showChatStatus | boolean | (Optional) Displays the chat connection status indicator | false |
showOnlineUsersTab | boolean | (Optional) Displays users tab with the list of online users | false |
hideNewChatButton | boolean | (Optional) Allows to hide the chat creation button | false |
hideNewUserChatOption | boolean | (Optional) Allows to hide the New Message option in New Chat dropdown | false |
hideNewGroupChatOption | boolean | (Optional) Allows to hide the New Group option in New Chat dropdown | false |
muted | boolean | (Optional) Mutes or unmutes notifications and sounds | false |
showNotifications | boolean | (Optional) Allows receiving browser notifications | false |
playSound | boolean | (Optional) Enables or disables playing sound on incoming messages | true |
webPushNotifications | boolean | (Optional) Allows receiving browser push notifications | false |
webPushVapidPublicKey | string | (Optional) Vapid Public Key for push notifications | false |
serviceWorkerPath | string | (Optional) Path to service worker for push notifications | false |
attachmentsAccept | string | (Optional) This prop sets to the accept attribute in HTML | ”/“ |
enableCalls | boolean | (Optional) Enables the calls feature | false |
enableUserStatuses | boolean | (Optional) Enable user statuses, such as “Available”, “Busy” and “Away” | false |
enableLastSeen | boolean | (Optional) Displays green dot on user avatar in chats list when user is online and last seen information on chat header | false |
enableContentReporting | boolean | (Optional) Enable reporting bad content feature (will show Report button in user profile) | false |
enableBlockList | boolean | (Optional) Enable block user feature (will show Block button in user profile) | false |
enableOnlineUsersBadge | boolean | (Optional) Displays online users badge on chat widget button | false |
getOnlineUsersInterval | number | (Optional) Allows to set how frequently the badge should be updated, in seconds. Min is 30 seconds | 300 |
enableUrlPreview | boolean | (Optional) Allows to unfurl a link once posted in chat | false |
limitUrlsPreviews | number | (Optional) Allows to set maximum displayed URLs preview in single message. Max is 5 | 1 |
quickActions | object | (Optional) Configuration for quick actions dialog. See QuickActions | |
defaultChat | object | (Optional) Force widget to open particular chat | |
onUnreadCountChange | (count: boolean) => void | (Optional) Callback function to get update about unread messages count | |
onOnlineUsersCountChange | (count: boolean) => void | (Optional) Callback function to get update about online users count | |
onOpenChange | (open: boolean) => void | (Optional) Callback function to get update about chat widget visibility |
Quick Actions
Section titled “Quick Actions”Prop Name | Type | Description | Default Value |
---|---|---|---|
title | string | (Optional) Title for the quick actions section | |
description | string | (Optional) Description for the quick actions | |
actions | string[] | List of action strings |
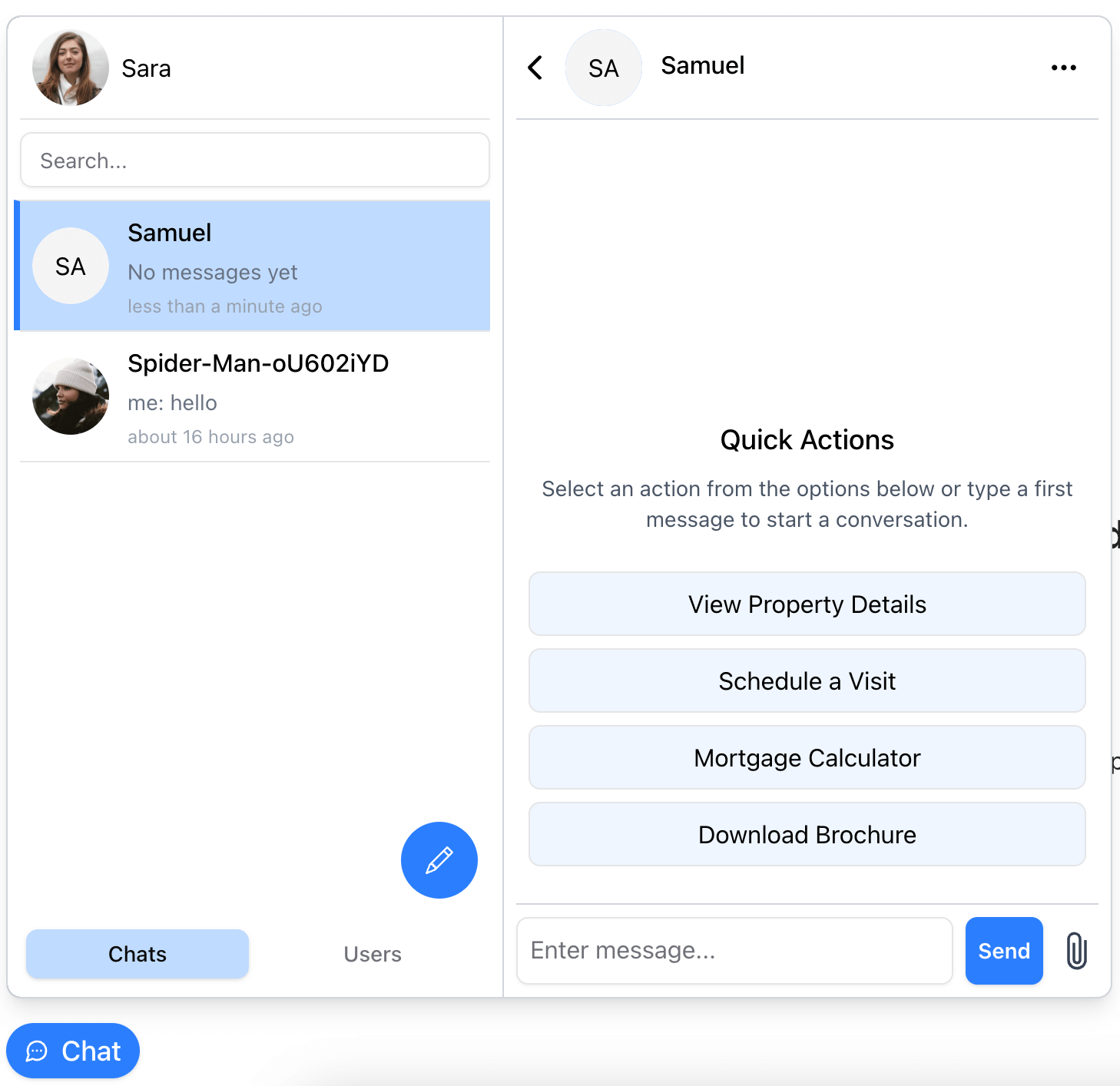
Supported Translations
Section titled “Supported Translations”Language Code | Language |
---|---|
en | English |
el | Greek |
ua | Ukrainian |
Default Chat
Section titled “Default Chat”Prop Name | Type | Description | Default Value |
---|---|---|---|
id | string | A key that will be used to identify the chat | |
opponentUserId | string | User id to create chat with. Must be a User Id from your system, similar to userId prop | |
type | string | Type of chat to create if not exist: 1on1 or group | |
name | string | Name of new chat | |
metadata | map | New chat metadata |
Push notifications
Section titled “Push notifications”Push notifications allow to receive a message while the browser tab with widget is closed.
For push notifications to work it need to do the following:
- pass
webPushNotifications: true
prop - go to ConnectyCube Dashboard, Push Notifications, Credentials, WEBPUSH NOTIFICATIONS and fill all the required fields (Subject, PublicKey, PrivateKey)
- for
webPushVapidPublicKey
prop to set the same PublicKey which you set in ConnectyCube Dashboard. - create a service worker and provide path to it via
serviceWorkerPath
prop. See chat widget code samples as a reference.
Recipes
Section titled “Recipes”Split view
Section titled “Split view”<ConnectyCubeChatWidget ... splitView={true}/>
const props = { ... splitView: true,};
Single view
Section titled “Single view”<ConnectyCubeChatWidget ... splitView={false}/>
const props = { ... splitView: false,};
Embedded view
Section titled “Embedded view”<ConnectyCubeChatWidget ... embedView={true}/>
const props = { ... embedView: true,};
Display Chat button bottom left
Section titled “Display Chat button bottom left”<ConnectyCubeChatWidget ... buttonStyle={{"left": "0.5rem", "right": "auto"}} portalStyle={{"left": "0.5rem", "right": "auto"}}/>
const props = { ... buttonStyle: {"left": "0.5rem", "right": "auto"} portalStyle: {"left": "0.5rem", "right": "auto"},};
Open widget on custom button
Section titled “Open widget on custom button”<ConnectyCubeChatWidget ... open={true}/>
const openChatBtn = document.getElementById('openChatButton');openChatBtn.addEventListener('click', () => { window.ConnectyCubeChatWidget.toggle()});
Have an issue?
Section titled “Have an issue?”Join our Discord for quick answers to your questions
Changelog
Section titled “Changelog”https://github.com/ConnectyCube/connectycube-chat-widget-samples/blob/main/CHANGELOG.md